Other Parts Discussed in Thread: ADS1260
Hello. I am designing a load cell with ADS1235 as the ADC with a pre-amplification system using AD8557 as OPAMPS, and STM32F405RGT6 as uC for this system.
I can successfully measure and change gain settings using firmware libraries provided by TI for the ADS1235.
For my application, I need to calculate angle by measuring forces in 2 separate channels (X and Y), and the taring process is very critical to guarantee that the zeros are correctly found and I don't have any pre-charges on any of the channels before the calibration to successfully find the load coefficients.
I designed a taring function that looks like this:
Before taring, with ADC gain set to 1 and registers are set as follows, I get a signal like this
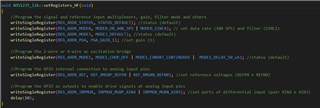
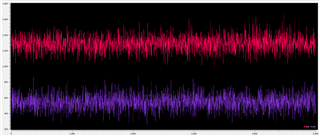
after I execute a tare using 1000 samples as input, the signal does this:
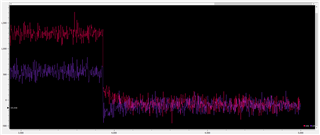
Am I calling the function in a wrong way that maybe is electrically destabilizing the ADC so during the tare() function the readings are not accurate? Is there any way in which I may do this differently? I kindly wait for your response.
Thank you very much for the support,
João